Unit 3 LA 1
For the mini-project of LA 1 the assignment was to create a wallpaper and vary this so you have 3 versions. WE had to use for-loops to play with size and color. I think it was a fun yet easy assignment to do, although I had more struggles with getting a random function in there. Now I’ll show you my 3 designs.
The designs
1.

let h, s, b;
function setup() {
createCanvas(800, 450);
colorMode(HSB);
let h = random(360);
let s = random(360);
let b = random(360);
background('#ffffff');
}
function draw() {
for (var y = 0; y <= height; y += 40) {
for (var x = 0; x <= width; x += 40){
noStroke();
fill(x /10, y/3, 100);
ellipse(x, y, 40, 40);
stroke('#ffffff');
strokeWeight(2);
fill(x / 10 + 280, y / 3 + 180, 100);
ellipse(x ,y, x / 20, x / 20);
}
}
}
2.
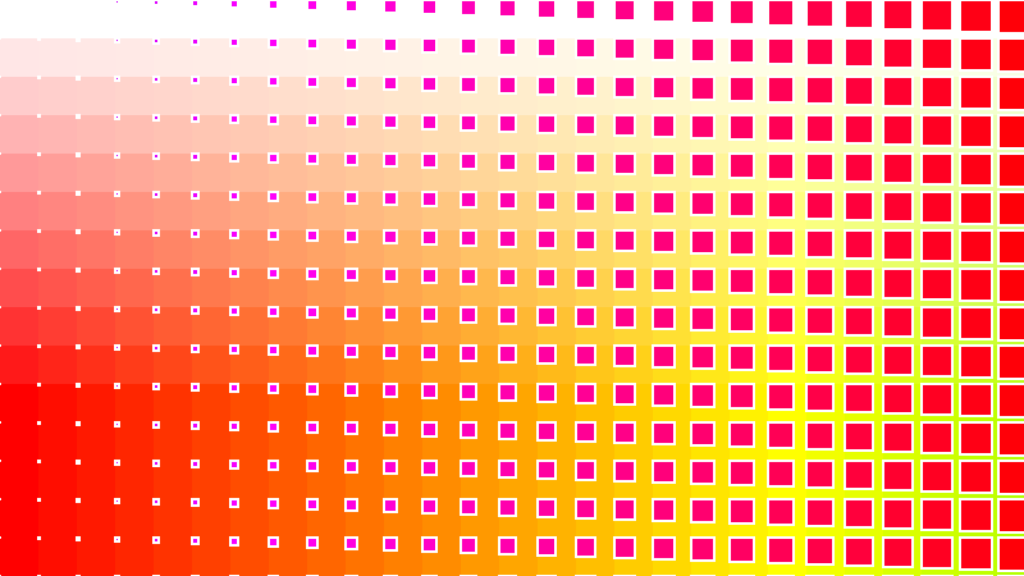
let h, s, b;
function setup() {
createCanvas(800, 450);
colorMode(HSB);
let h = random(360);
let s = random(360);
let b = random(360);
background('#ffffff');
}
function draw() {
for (var y = 0; y <= height; y += 30) {
for (var x = 0; x <= width; x += 30){
noStroke();
fill(x /10, y/3, 100);
rect(x, y, 30, 30);
stroke('#ffffff');
strokeWeight(2);
fill(x / 10 + 280, y / 3 + 180, 100);
rect(x ,y, x / 30, x / 30);
}
}
}
3.
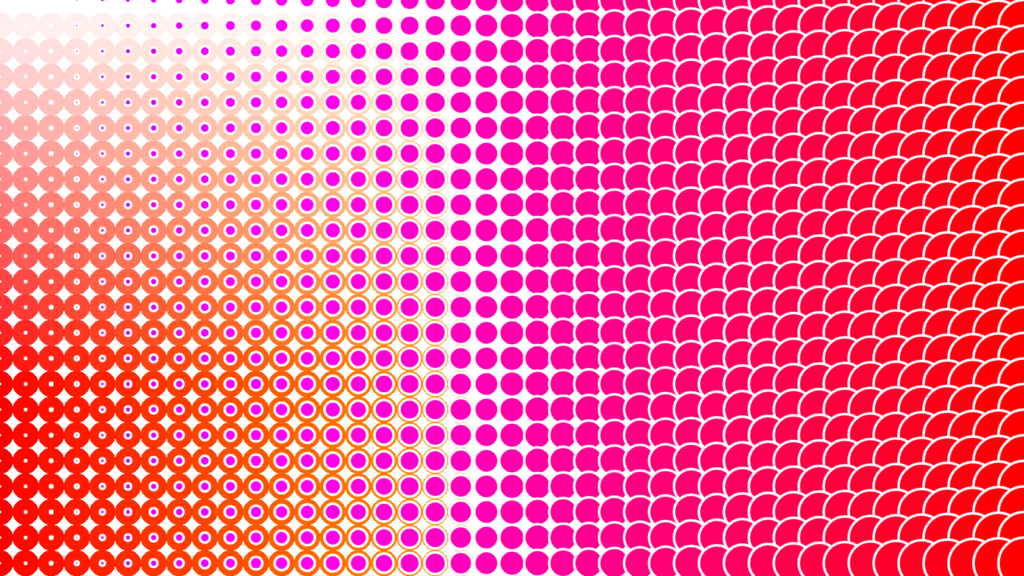
let h, s, b;
function setup() {
createCanvas(800, 450);
colorMode(HSB);
background('#ffffff');
}
function draw() {
for (var y = 0; y <= height; y += 20) {
for (var x = 0; x <= width; x += 20){
noStroke();
fill(x /10, y/3, 100);
ellipse(x, y, 20, 20);
stroke('#ffffff');
strokeWeight(2);
fill(x / 10 + 280, y / 3 + 180, 100);
ellipse(x ,y, x / 20, x / 20);
}
}
}
In comparison:


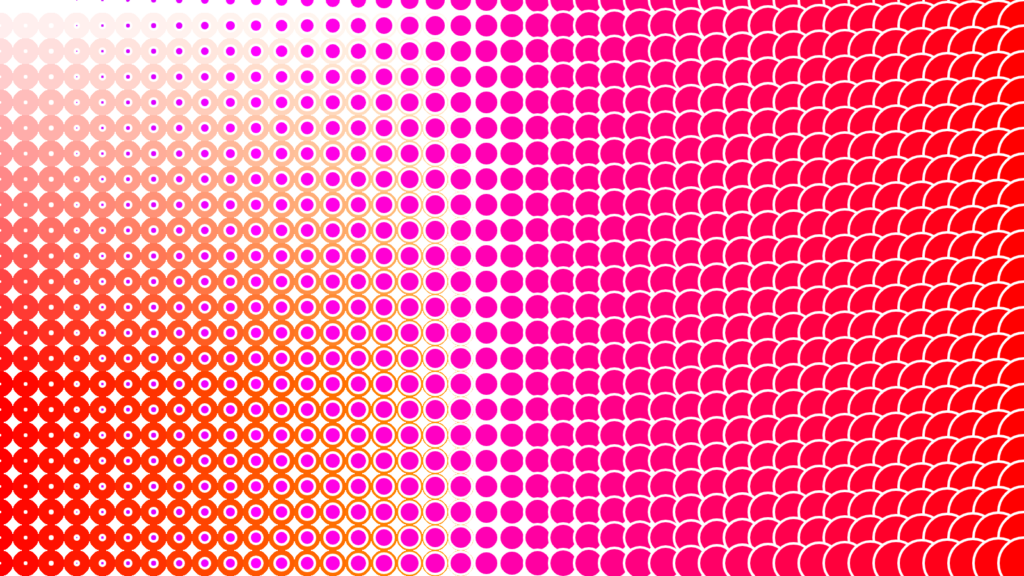
Writing prompt
I think these wallpapers should be added to windows, because they are really funky and even mesmerizing to look at. The second design even has a fun illusion included and the colors of the design are bright and modern. Personally I really like the sunset colors and that is why these designs mean something to me. The pattern I created is something I’ve always liked and wanted to explore and see what I could do and now I finally did.
Random?
In the end I decided to try the random wallpaper again and this time I succeeded. I made the amount of of the shapes random. Hereby aslo their size has become random.
let h, s, b, r, f;
function setup() {
createCanvas(800, 450);
colorMode(HSB);
let h = random(360);
let s = random(360);
let b = random(360);
background('#ffffff');
}
function draw() {
let r = random(10, 60);
for (var y = 0; y <= height; y += r) {
for (var x = 0; x <= width; x += r){
noStroke();
fill(x /10, y/3, 100);
ellipse(x, y, r, r);
stroke('#ffffff');
strokeWeight(2);
fill(x / 10 + 280, y / 3 + 180, 100);
ellipse(x ,y, x / 40, x / 40);
}
}
noLoop();
}