Lightswitch game
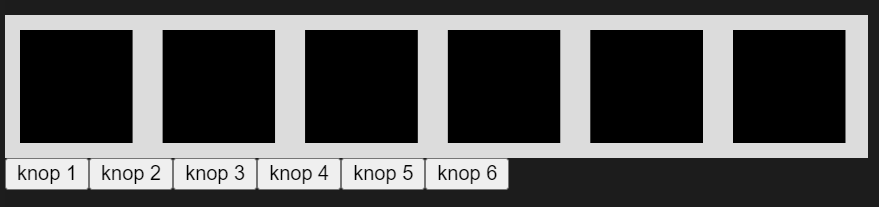
https://editor.p5js.org/SuperiorCrane1/sketches/LgGHhZRuM
//lampstatuses
var lamp1status = false
var lamp2status = false
var lamp3status = false
var lamp4status = false
var lamp5status = false
var lamp6status = false
//lamp color
var activeColor = [255, 246, 69]
var inactiveColor = [0, 0, 0]
var lamp1color = inactiveColor
var lamp2color = inactiveColor
var lamp3color = inactiveColor
var lamp4color = inactiveColor
var lamp5color = inactiveColor
var lamp6color = inactiveColor
//buttons
var button1
var button2
var button3
var button4
var button5
var button6
function setup() {
createCanvas(575, 95);
button1 = createButton('knop 1')
button1 .mousePressed(() => {
lamp3status =! lamp3status
lamp6status =! lamp6status
})
button2 = createButton('knop 2')
button2 .mousePressed(() => {
lamp3status =! lamp3status
lamp4status =! lamp4status
})
button3 = createButton('knop 3')
button3 .mousePressed(() => {
lamp1status =! lamp1status
})
button4 = createButton('knop 4')
button4 .mousePressed(() => {
lamp5status =! lamp5status
})
button5 = createButton('knop 5')
button5 .mousePressed(() => {
lamp3status =! lamp3status
})
button6 = createButton('knop 6')
button6 .mousePressed(() => {
lamp1status =! lamp1status
lamp2status =! lamp2status
})
}
function draw() {
background(220);
noStroke()
fill(lamp1color)
rect(10, 10, 75) //lamp 1
fill(lamp2color)
rect (105, 10, 75)
fill(lamp3color)
rect(200, 10, 75)
fill(lamp4color)
rect(295, 10, 75)
fill(lamp5color)
rect(390, 10, 75)
fill(lamp6color)
rect(485, 10, 75)
//colorchanger
if(!lamp1status){
lamp1color = inactiveColor
} else {
lamp1color = activeColor
}
if(!lamp2status){
lamp2color = inactiveColor
} else {
lamp2color = activeColor
}
if(!lamp3status){
lamp3color = inactiveColor
} else {
lamp3color = activeColor
}
if(!lamp4status){
lamp4color = inactiveColor
} else {
lamp4color = activeColor
}
if(!lamp5status){
lamp5color = inactiveColor
} else {
lamp5color = activeColor
}
if(!lamp6status){
lamp6color = inactiveColor
} else {
lamp6color = activeColor
}
//win control
if(lamp1status && lamp2status && lamp3status && lamp4status && lamp5status && lamp6status){
textSize(50)
textAlign(CENTER, CENTER)
fill('black')
text(' jE hEbT gEwOnNeN ', width/2, height/2)
}
}
Paint in P5JS
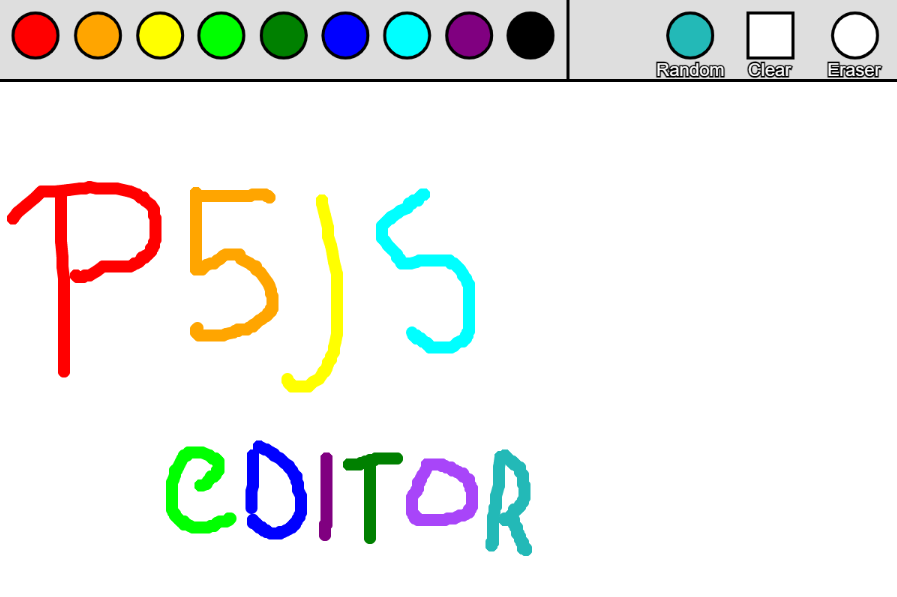
https://editor.p5js.org/SuperiorCrane1/sketches/vZ5RFyxXC
var toolColor = "black";
var d;
function setup() {
createCanvas(600, 400);
strokeWeight(2);
smooth();
background(255);
//start color
r = 0;
g = 0;
b = 0;
}
function draw() {
fill(222);
rect(0, 0, 380, 55);
fill(222);
rect(380, 0, 380, 55);
strokeWeight(8);
stroke(toolColor);
if (mouseIsPressed) line(pmouseX, pmouseY, mouseX, mouseY);
stroke("black");
fill(0);
if(toolColor === 'red'){
ellipseMode(RADIUS);
fill(0);
ellipse(25, 25, 18, 18);
ellipseMode(CENTER);
} else if(toolColor === 'orange'){
ellipseMode(RADIUS);
fill(0);
ellipse(66.5, 25, 18, 18);
ellipseMode(CENTER);
} else if(toolColor === 'yellow'){
ellipseMode(RADIUS);
fill(0);
ellipse(108, 25, 18, 18);
ellipseMode(CENTER);
} else if(toolColor === 'lime'){
ellipseMode(RADIUS);
fill(0);
ellipse(108 + 41.1, 25, 18, 18);
ellipseMode(CENTER);
} else if(toolColor === 'green'){
ellipseMode(RADIUS);
fill(0);
ellipse(190.2, 25, 18, 18);
ellipseMode(CENTER);
} else if(toolColor === 'blue'){
ellipseMode(RADIUS);
fill(0);
ellipse(190.2 + 41.1, 25, 18, 18);
ellipseMode(CENTER);
} else if(toolColor === 'aqua'){
ellipseMode(RADIUS);
fill(0);
ellipse(190.2 + 82.2, 25, 18, 18);
ellipseMode(CENTER);
} else if(toolColor === 'purple'){
ellipseMode(RADIUS);
fill(0);
ellipse(313.5, 25, 18, 18);
ellipseMode(CENTER);
} else if(toolColor === 'black'){
ellipseMode(RADIUS);
fill(0);
ellipse(190.2 + 82.2 + 82.2, 25, 18, 18);
ellipseMode(CENTER);
} else if(toolColor === 'white'){
ellipseMode(RADIUS);
fill(0);
ellipse(width/1.05, 25, 18, 18);
ellipseMode(CENTER);
}
//----------Red------------
strokeWeight(2);
if (mouseIsPressed &&
mouseX > width / 24 - 20 &&
mouseX < width / 24 + 20 &&
mouseY > height / 16 - 20 &&
mouseY < height / 16 + 20
) {
ellipseMode(RADIUS);
fill(0);
ellipse(25, 25, 18, 18);
ellipseMode(CENTER);
fill('red');
toolColor = "red";
} else {
fill('red');
}
ellipse(width / 24, height / 16, 30, 30);
//----------Orange----------
if (mouseIsPressed &&
mouseX > width / 9 - 20 &&
mouseX < width / 9 + 20 &&
mouseY > height / 16 - 20 &&
mouseY < height / 16 + 20
) {
ellipseMode(RADIUS);
fill(0);
ellipse(66.5, 25, 18, 18);
ellipseMode(CENTER);
fill('orange');
toolColor = "orange";
} else {
fill('orange');
}
ellipse(width / 9, height / 16, 30, 30);
//----------Yellow------------
if (mouseIsPressed &&
mouseX > width / 5.55 - 20 &&
mouseX < width / 5.55 + 20 &&
mouseY > height / 16 - 20 &&
mouseY < height / 16 + 20
) {
ellipseMode(RADIUS);
fill(0);
ellipse(108, 25, 18, 18);
ellipseMode(CENTER);
fill('yellow');
toolColor = "yellow";
} else {
fill('yellow');
}
ellipse(width / 5.55, height / 16, 30, 30);
//---------------Lime----------------
if (mouseIsPressed &&
mouseX > width / 4.03 - 20 &&
mouseX < width / 4.03 + 20 &&
mouseY > height / 16 - 20 &&
mouseY < height / 16 + 20
) {
ellipseMode(RADIUS);
fill(0);
ellipse(108 + 41.1, 25, 18, 18);
ellipseMode(CENTER);
fill('lime');
toolColor = "lime";
} else {
fill('lime');
}
ellipse(width / 4.03, height / 16, 30, 30);
//----------green--------------
if (mouseIsPressed &&
mouseX > width / 3.15 - 20 &&
mouseX < width / 3.15 + 20 &&
mouseY > height / 16 - 20 &&
mouseY < height / 16 + 20
) {
ellipseMode(RADIUS);
fill(0);
ellipse(190.2, 25, 18, 18);
ellipseMode(CENTER);
fill('green');
toolColor = "green";
} else {
fill('green');
}
ellipse(width / 3.15, height / 16, 30, 30);
//------------------blue-----------------
if (mouseIsPressed &&
mouseX > width / 2.59 - 20 &&
mouseX < width / 2.59 + 20 &&
mouseY > height / 16 - 20 &&
mouseY < height / 16 + 20
) {
ellipseMode(RADIUS);
fill(0);
ellipse(190.2 + 41.1, 25, 18, 18);
ellipseMode(CENTER);
fill('blue');
toolColor = "blue";
} else {
fill('blue');
}
ellipse(width / 2.59, height / 16, 30, 30);
//-----------aqua-----------
if (mouseIsPressed &&
mouseX > width / 2.2 - 20 &&
mouseX < width / 2.2 + 20 &&
mouseY > height / 16 - 20 &&
mouseY < height / 16 + 20
) {
ellipseMode(RADIUS);
fill(0);
ellipse(190.2 + 82.2, 25, 18, 18);
ellipseMode(CENTER);
fill('aqua');
toolColor = "aqua";
} else {
fill('aqua');
}
ellipse(width / 2.2, height / 16, 30, 30);
//-------------Purple-------------
if (mouseIsPressed &&
mouseX > width / 1.91 - 20 &&
mouseX < width / 1.91 + 20 &&
mouseY > height / 16 - 20 &&
mouseY < height / 16 + 20
) {
ellipseMode(RADIUS);
fill(0);
ellipse(313.5, 25, 18, 18);
ellipseMode(CENTER);
fill('purple');
toolColor = "purple";
} else {
fill('purple');
}
ellipse(width / 1.91, height / 16, 30, 30);
//------------Black----------------
if (mouseIsPressed &&
mouseX > width / 1.69 - 20 &&
mouseX < width / 1.69 + 20 &&
mouseY > height / 16 - 20 &&
mouseY < height / 16 + 20
) {
ellipseMode(RADIUS);
fill(0);
ellipse(190.2 + 82.2 + 82.2, 25, 18, 18);
ellipseMode(CENTER);
fill(0);
toolColor = "black";
} else {
fill(0);
}
ellipse(width / 1.69, height / 16, 30, 30);
//-----------------eraser-------------
if (mouseIsPressed &&
mouseX > width / 1.05 - 20 &&
mouseX < width / 1.05 + 20 &&
mouseY > height / 16 - 20 &&
mouseY < height / 16 + 20
) {
ellipseMode(RADIUS);
fill(0);
ellipse(width/1.05, 25, 18, 18);
ellipseMode(CENTER);
fill('white');
toolColor = "white";
} else {
fill('white');
}
ellipse(width / 1.05, height / 16, 30, 30);
//--------clear----------
if (mouseIsPressed &&
mouseX > width / 1.20 - 20 &&
mouseX < width / 1.20 + 20 &&
mouseY > height / 16 - 20 &&
mouseY < height / 16 + 20
) {
rectMode(CORNER)
fill('white')
rect(0, 55, 600, 4500)
} else {
fill('white');
}
rect(width / 1.20, 10, 30, 30);
//------------random color----------
if (mouseIsPressed &&
mouseX > width / 1.30 - 20 &&
mouseX < width / 1.30 + 20 &&
mouseY > height / 16 - 20 &&
mouseY < height / 16 + 20
) {
let r = random(0, 255)
let g = random(0, 255)
let b = random(0, 255)
toolColor = [r, g, b]
}
fill(toolColor)
ellipse(width / 1.30, height / 16, 30, 30);
fill(255)
//---------text for clear--------
text("Clear", width/1.20, 52)
//--------text for eraser--------
text("Eraser", width/1.085, 52)
//----------text for random color------------
text("Random ", width/1.367, 52)
}