Please Touch Everything
The Code – The Fullscreen Game – The grade
This time we got the assignment to make a game, specifically a game where you get multiple buttons and multiple light with the objective to turn every light on. So naturally i made a game where a nuclear bomb goes off once the correct “code” in entered.
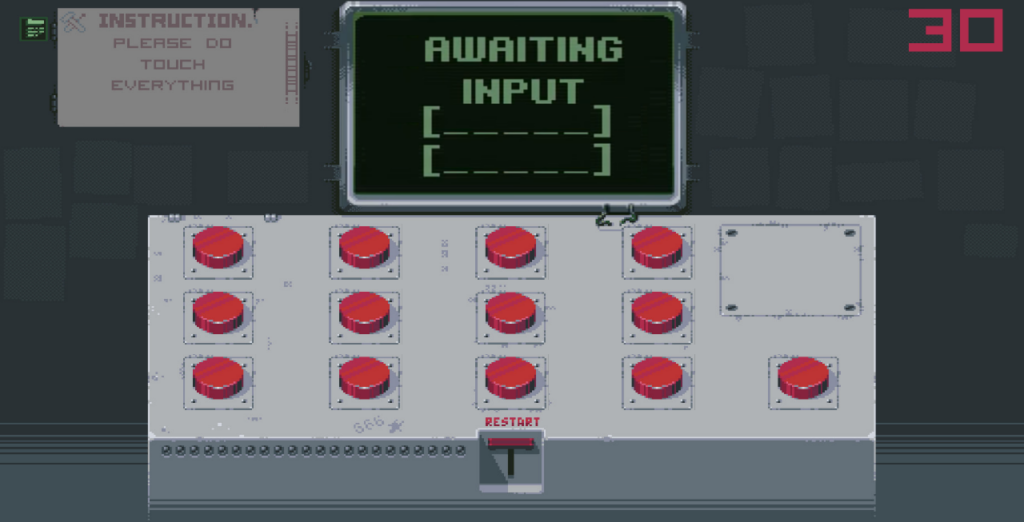
this is the screen you are greeted with. it is very, very heavily stolen from a game called “Please Don’t Touch Anything” this game puts you in a room in front of a panel with a single red button, after a bit of dialogue from your colleague warning you to Please Don’t Touch Anything while they go to get a cup of coffee you are given free reign over the pannel with the big ominous red button just begging to be pushed. it is a great game with a ton of different fun endings and a simplistic yet wonderful art style. so i had a base (the game), an idea (the explosion) and an objective (button make stuff go boom). A great load of screenshots with many attempts at photoshopping while not being able to run photoshop later i made a couple of sprites so that i could use the images i had taken from the game and they would fit my very specific needs.
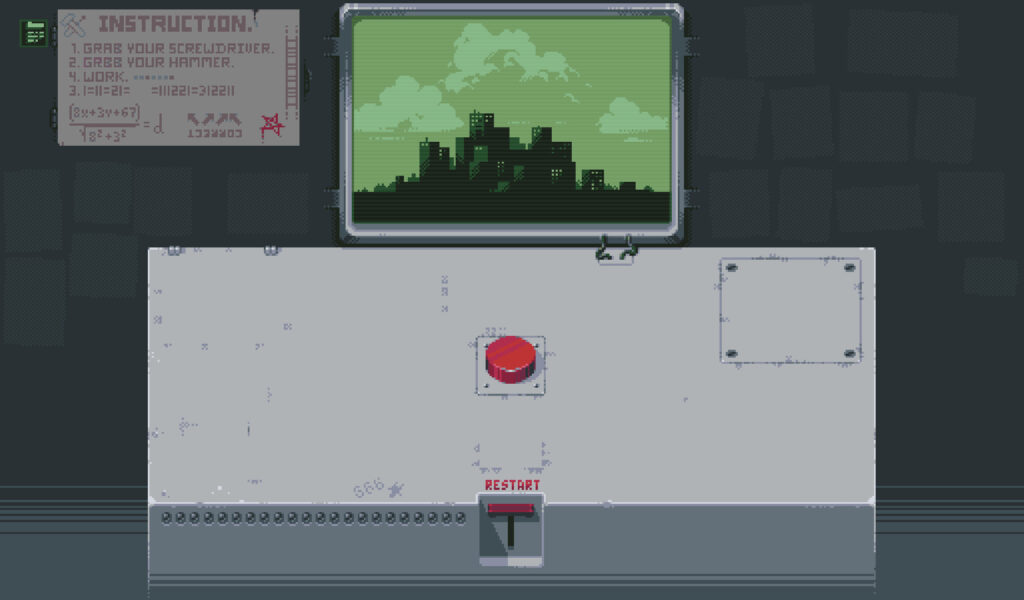
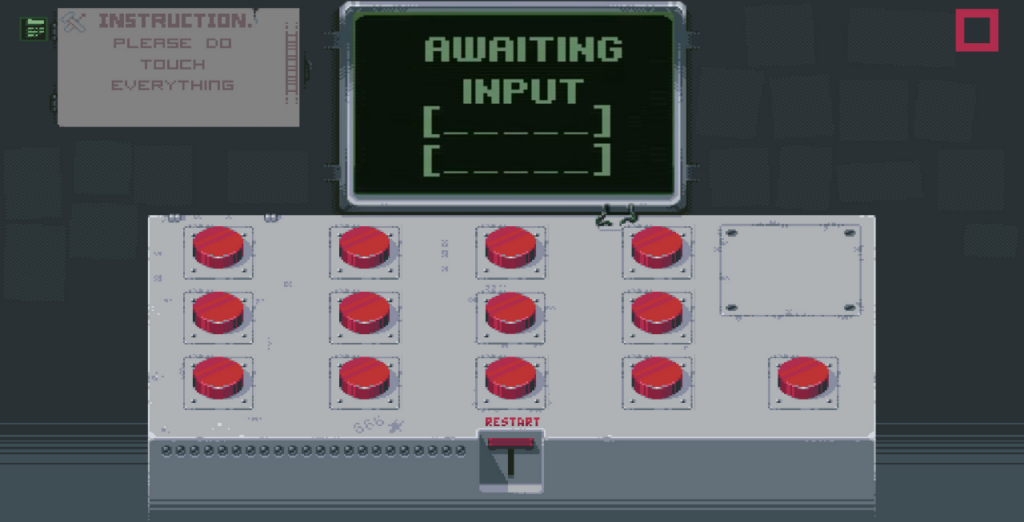
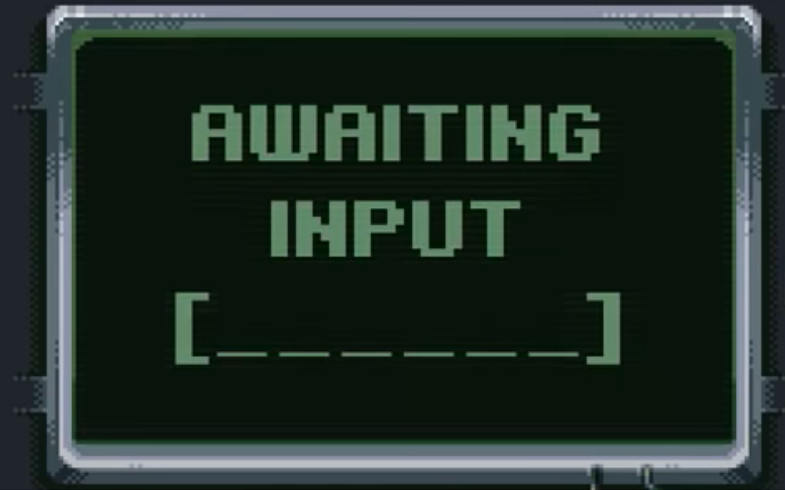
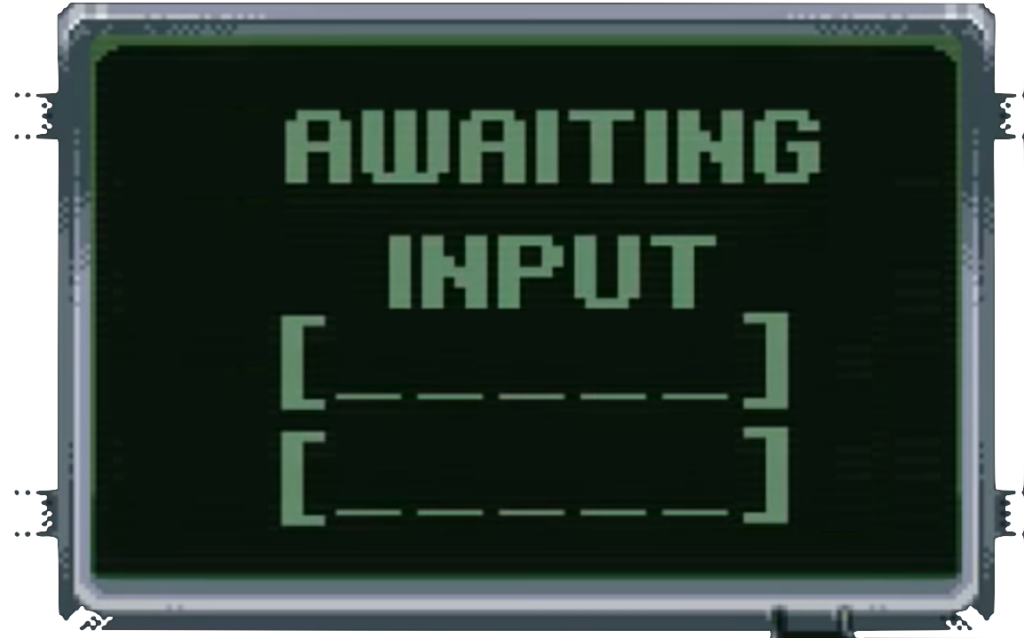
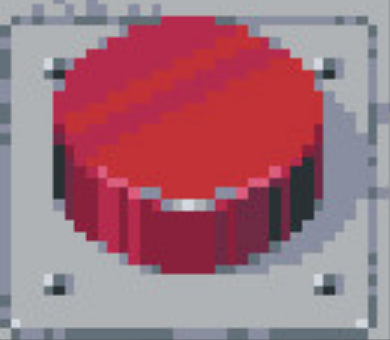
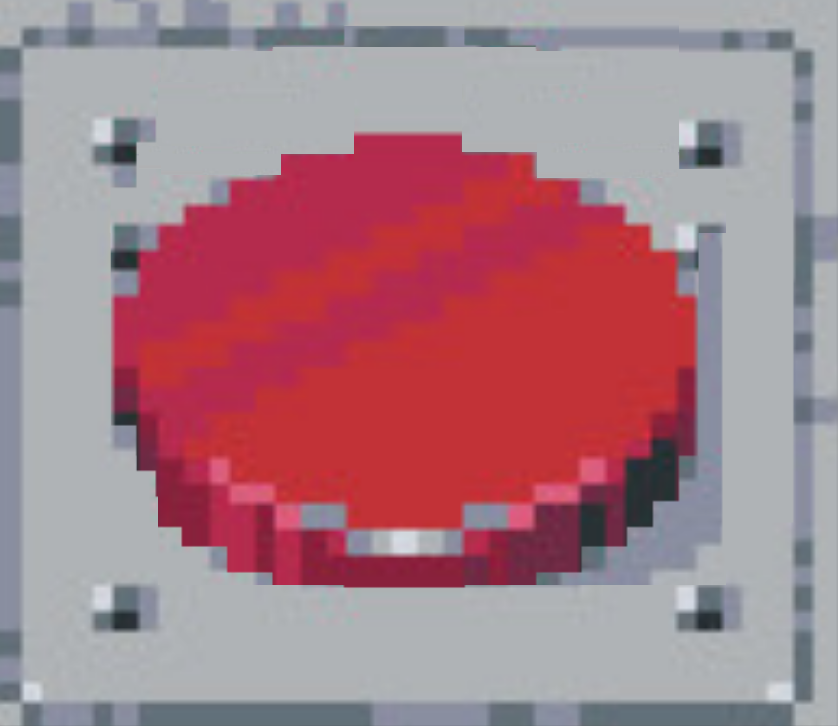
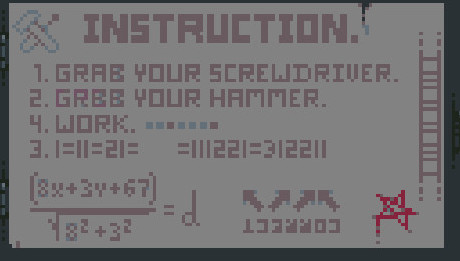
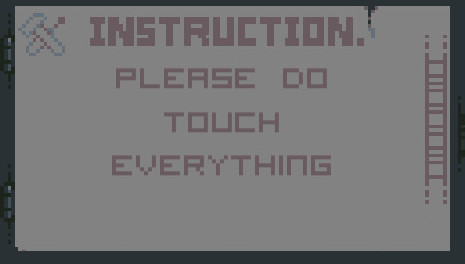
Now that i painstakingly crafted an interface only the easy part was left, to actually make a working functioning thing.
The logic behind the the game is fairly simple. every button is a boolean variable (A – M) it can either be true or false, a 1 or 0. true is on and false is off. Now every light needs its own boolean variable (X0-X9) and a specific string of buttons that must be true or must be false to make the light turn on. this makes the light a boolean function of the buttons.
X0 = (H && !F && G && E && D) || (!A && C && F) || (L && !K);
X1 = (B && A && G && !J && !L) || ((!B || !A) && F && J) || (!J && I);
X2 = (G && I && !M && E && D) || (!I && !D && J && L) || A;
X3 = (A && B && I && D && !F) || (!E && !G && !H && M && C) || K;
X4 = (D && E && !C && A && G && H) || (!(A || H) && L) || (!G && H);
X5 = (!K && G && A && !M && H && B) || ((!E || !B) && M && L);
X6 = (D && A && B && E && G && D) || (!(D || B || H) && M && L);
X7 = (!J && A && E && I && D) || (!I && C && M) || F || B;
X8 = (B && H && A && E && !C) || (!E && !G && L && K) || (J && L);
X9 = (G && A && D && E) || (!D && L && F && C);
fin = B && I && G && H && E && A && D && !C && !F && !J && !K && !L && !M;
Lucky there are things wonderful things called Boolean Operators. there are a few of these operators, the ones i used where; || known as OR, && known as AND, and ! known as NOT. with the power of these three operators and the liberal usage of bracket you can create multiple combinations for example
X = A || B | if A is true OR B is true, X is true |
X = A && B | if A is true AND B is true, X is true |
X = !A || B | if A is false OR B is true, X is true |
once you get a bit creative with it you can make some very specific button combinations to turn the lights on or off. you can also choose a few buttons that must be true if you want all the light to go on. this makes it easy to built in and make sure there is an actual solution. the code word for my game is *REDACTED*, if you turn all those buttons on you win the game.
with the logic done the next step was to connect the brains to the brawn, this wasn’t to difficult however i am over the top and decided it would be fun to have 10 lights and 13 buttons. it was a lot of repetitive typing and the copy paste function was truly a life saviour.
function mouseClicked() {
//A
if (
mouseX >= (width / 28) * 5 &&
mouseX <= (width / 28) * 7 &&
mouseY >= (height / 56) * 24 &&
mouseY <= (height / 56) * 30
) {
if (A) {
A = false;
} else {
A = true;
}
this bit of code is essentially a switch, it toggles the buttons. if you click your mouse whilst in those specific parameter it checks to see if the button is on, if it is it turns it off if it isn’t it turns the button on. this repeated 12 more times, all with individual parameters gives you a whole bunch of switches to flip. the only issue is that nothing visually changes so it is difficult to remember what buttons have and havent already been toggled this isn’t exactly user friendly as this is a light switch game, and not a guessing game. so we need a visual representation and response to the button being pressed
if (!A) {
image(
button,
(width / 28) * 5,
(height / 56) * 24,
(width / 28) * 2,
(height / 28) * 3
);
} else {
image(
press,
(width / 28) * 5,
(height / 56) * 24,
(width / 28) * 2,
(height / 28) * 3
);
}
At the start of the file i declared a few images, two of which are used in this small bit of code, one called button, which just shows a red button. And one called press, this image shows the same red button but lowered as if it has been pressed (hence the name). This bit of code checkes if A is false, if A is false it shows the image of the full red button if A is true and has there for been activated the image shows the pressed button. this gives the user feedback to their input and makes the game more compleet.
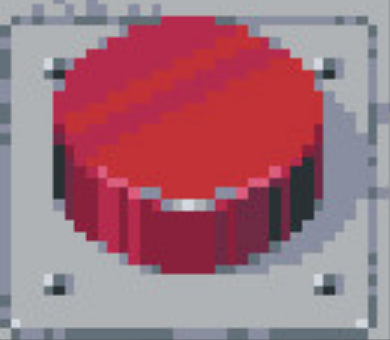
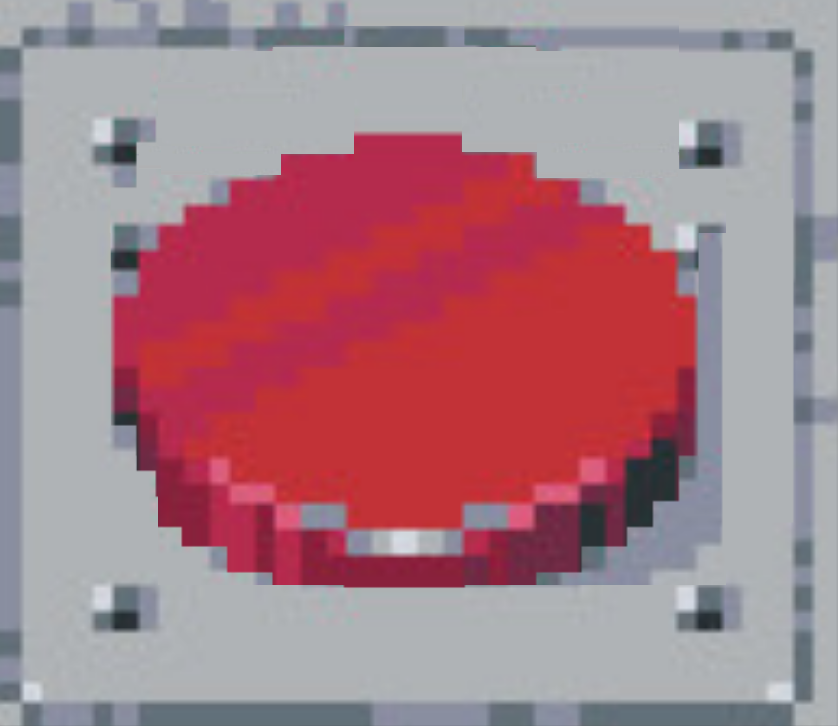
the next step in the process is to have the light turned on once the right buttons are pressed. this code is mostly finished as the boolean function checks whether or not a light should turn on or not, The output of the boolean functions can be used to turn on and off the image representing the light
{
if (X0) {
image(
input,
(width / 448) * 194,
(height / 448) * 96,
(width / 448) * 12,
(height / 224) * 10
);
}
If X0 is true the image of the first light is displayed, if X0 is false the place of the image will remain empty
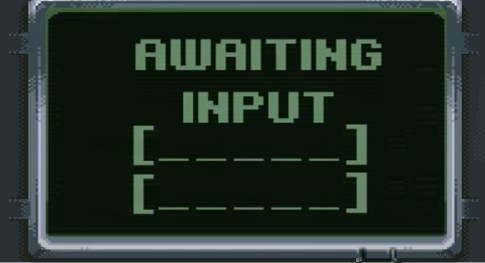
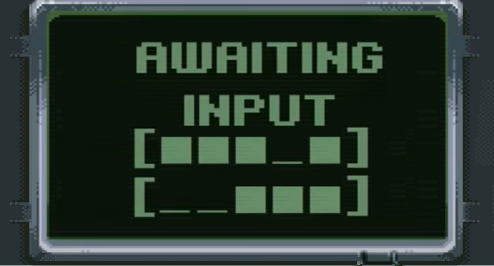
Now there are working buttons, and working lights, one might say that the game is now finished.
They would be wrong.
See when i started this project i saw a tiny city been blown to bits and i will not rest till every last bit of that city is gone fore you can not have a room with big red buttons without a nuke. Thats like having crumpets without butter or cheese without toast, it can not, and more importantly, should not be done. This game was going to go out with a bang.
To make the game go boom i made a nother boolean function. this function dictates that if all the buttons from the code word which is as you know *REDACTED*, are pressed and all the other buttons are not pressed something magical happens. this magical thing is a nother line of code very similar to the ones seen before however the image is an image of an 16 bit nuke on a tiny screen on your own screen… wahoo. at least this tiny mushroom cloud does not come alone. it comes paired with a truly rattling sound named “splojen” cause typing explosion is to much work and i could not be bothered to find out how to spell it.
if (fin) {
image(
boom,
(width / 224) * 70,
0,
(width / 448) * 165,
(height / 224) * 92
);
}
if (fin) {
splojen.play();
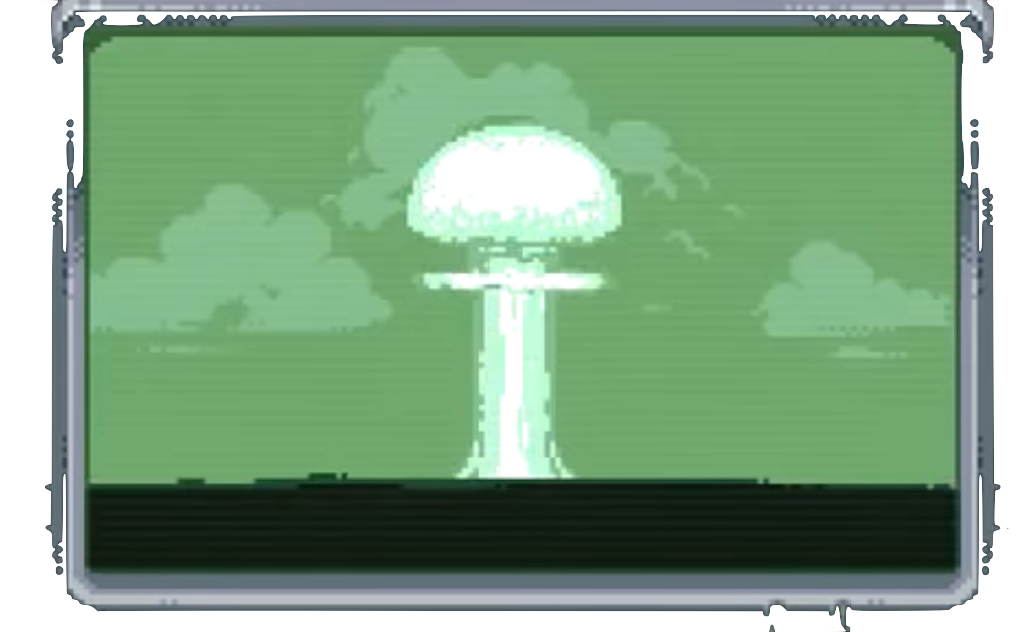
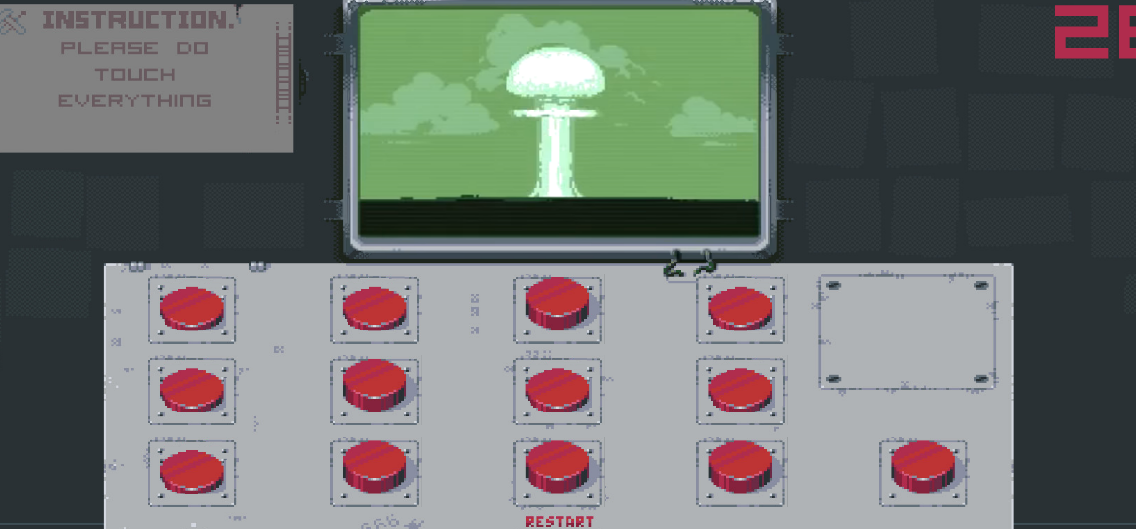
Now there are working buttons, working lights, and a truly spectacular ending. im still not finished though cause every game needs consequences and a bit of anxiety to keep things fresh. So i made a click counter. every click clicked with in the button area (prevents un intentional clicks from being counted) one is subtracted from the 30 in the top right corner. once the timer reaches zero game over
function mouseClicked() {
if (
mouseX >= (width / 28) * 5 &&
mouseX <= (width / 28) * 23 &&
mouseY >= (height / 56) * 24 &&
mouseY <= (height / 56) * 44 && !fin
) {
clicks--;
}
}
Every mouse click the location of the mouse is checked, if it falls into the parameters one is subtracted from the variable “clicked” which is defined as being 30 at the very start of the program
if (clicks < 0) {
clicks = 30;
}
textFont(visitor);
fill(345, 75, 69);
textSize(width / 12);
textAlign(CENTER, CENTER);
//displaying number of clicks
if (clicks < 10) {
text(clicks, (width / 48) * 46, height / 24);
} else {
text(clicks, (width / 48) * 45, height / 24);
}
this bit of code shows the counter displayed in the top right corner, i chose this font to fit in with the feeling of the game and the colour of the text is the same as that of the buttons. The counter moves slightly once the counter moves into single digits to prevent the numbers from clipping of screen or being to far from the corner. it also makes sure your amount of clicks doesn’t venture into the the negatives
if (clicks === 0) {
textFont(visitor);
fill(0, 0, 0);
rect(0, 0, width, height);
fill(345, 75, 69);
noStroke();
textSize(width / 6);
textAlign(CENTER, CENTER);
text("GAME OVER", width / 2, height / 2);
textSize(width / 10);
text("TRY AGAIN", width / 2, (height / 4) * 3);
}
this is the game over try again screen once the click counter reaches zero a black screen appears with the text “game over try again”
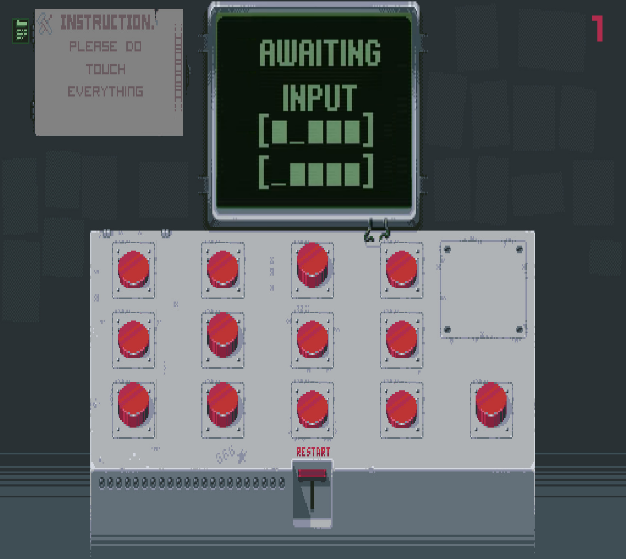
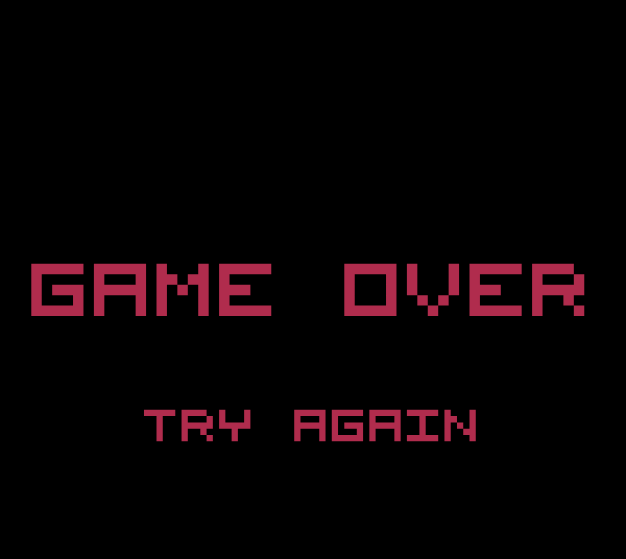
if (
clicks === -1 &&
mouseX >= width / 5 &&
mouseX <= (width / 5) * 4 &&
mouseY >= (height / 6) * 4 &&
mouseY <= (height / 6) * 5
) {
A = false;
B = false;
C = false;
D = false;
E = false;
F = false;
G = false;
H = false;
I = false;
J = false;
K = false;
L = false;
M = false;
clicks = 30;
}
once the game over try again screen shows up (clicks ===0) and you click (clicks === -1) on the try again text all buttons are set to false and the click counter resets to 30, this essentially means the game is reset
if (clicks > 0) {
//A
if (!A) }
all the coding to show the images for the buttons and lights only works when clicks is larger than 0 as images are shown on the top layer, so if you do not hide them they would still be visible once the game over try again try again screen shows.
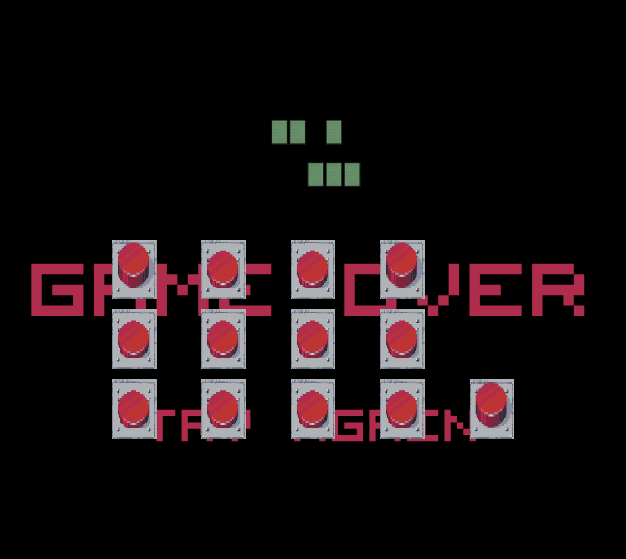
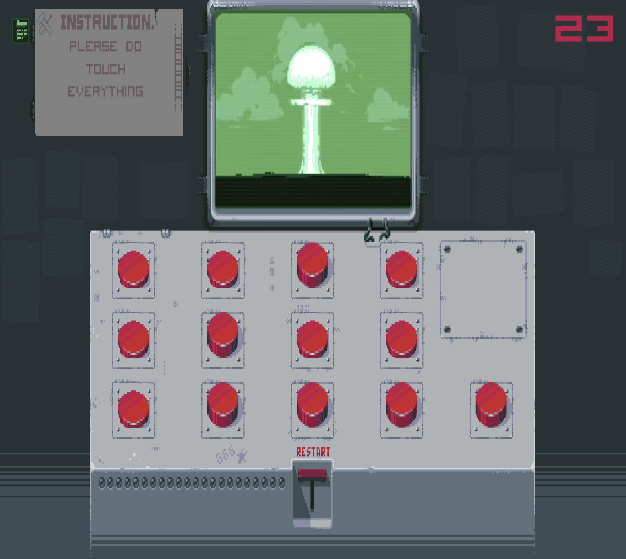
Working buttons, working lights, an ending, a counter and a game over screen?! surely that must be all right?
nooooop
The lovely game developers of “Please Don’t Touch Anything” were so kind to supply me with a restart button so i’d be a fool not to use it. no, the restart button does not restart the click counter. That would be the easy way out.
if (
mouseX >= (width / 28) * 13 &&
mouseX <= (width / 28) * 15 &&
mouseY >= (height / 56) * 44 &&
mouseY <= (height / 56) * 53
) {
A = false;
B = false;
C = false;
D = false;
E = false;
F = false;
G = false;
H = false;
I = false;
J = false;
K = false;
L = false;
M = false;
}
Thats it, That is the entire program, 714 lines of code neatly condensed into a measly 1853 word summery and explanation.
here you go, after reading through all of that nonsense you deserve it, the code is BIGHEAD