Microsoft Pain
The Code – The Fullscreen Game – The webpage – The grade
The final assignment for unit two was to recreated paint. so i started, as any sane person would, by looking at screenshots of paint and taking it into my photo editor to get all of the exact HSB colour values that the generic ms paint program uses for the brushes, background and toolbar, i figured that microsoft probably paid enough people enough money to make sure they chose the optimal colours so i should just use their hard work and research to my advantage.
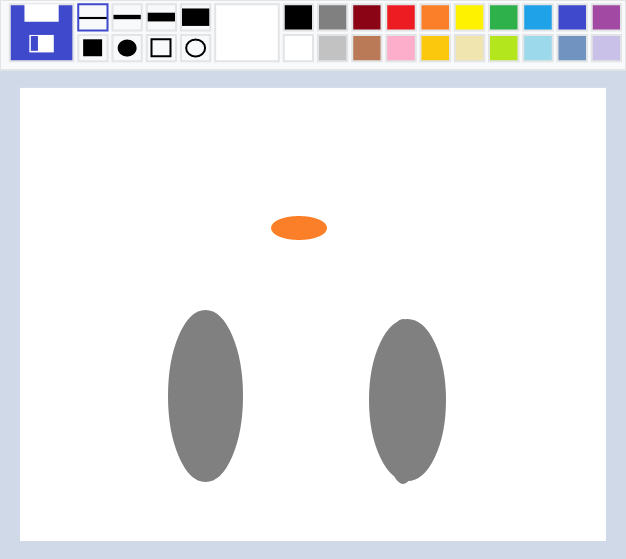
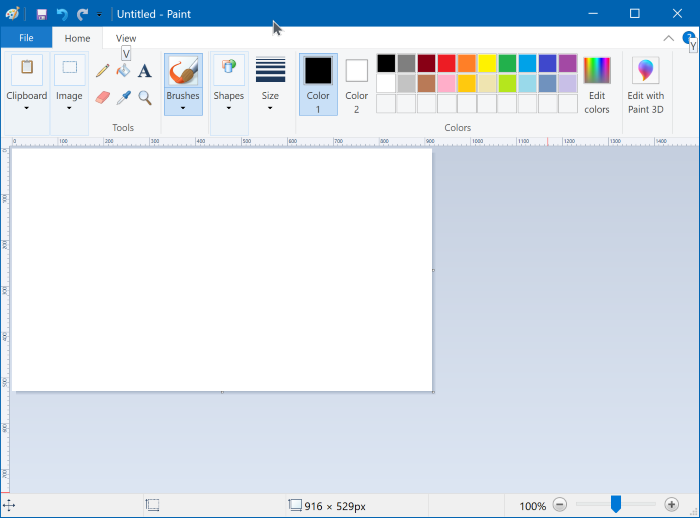
The journey started at the background or the canvas if you wil. all the borders and shapes are once again relative to the size of the screen. not only that but the colours of my program are selected to mirror the colours in paint. this bit of code creates a grey border around the white canvas
//border
{fill(215, 11, 91);
noStroke();
rect(0, 0, width / 32, height);
rect(0, 0, width, (height / 32) * 5);
rect((width / 32) * 31, 0, width, height);
rect(0, (height / 32) * 31, width, height);
}
this bit below is made tot be the header to the page
//Header
{
strokeWeight(2);
stroke(211, 1, 90);
fill(211, 1, 98);
rect(0, 0, width, height / 8);
}
so now we have a canvas which is complet and utterly useless without a place to select you tools which means i need to steal some more colour values from paint and make some squares and do some mind numbing maths to get everything to align
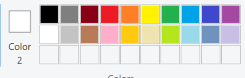

//black
fill(215, 11, 0);
rect((width / 128) * 58, height / 128, (width / 64) * 3, (height / 64) * 3);
this block of code was repeated 20 times with the exact colours and positions. each block of colour also has a grey border so it 20 blocks of colour with 20 underlaying grey squares
so, a canvas, funky colour next is the actual thing that makes the drawing happen… pretty important for a drawing program.
if (
recty === false &&
circy === false &&
erecty === false &&
ecircy === false
) {
stroke(x, y, z);
} else {
noStroke();
}
strokeWeight(w);
line(mouseX, mouseY, pmouseX, pmouseY);
}
this bit of code first makes sure that you don’t have a different tool selected, if you do have a different tool selected then it wil still draw the line but at least then the line in invisible. if no other tools are selected it will draw a line based on the mouses position with the assigned colour and strokewheight
//brush size
{
strokeWeight(2);
if (w === 4) {
stroke(236, 69, 80);
fill(214,41,100)
} else {
stroke(211, 1, 90);
noFill();
}
rect(
(width / 128) * 16,
height / 128,
(width / 64) * 3,
(height / 64) * 3
);
this bit helps the user see what stroke size they have selected, once a tool is selected either by clicking on it or using the number keys 1-4, the square of the corresponding tool turns blue. this also counts fore the square or ellipse draw tools, the big box to the right shows the current selected colour, if you click this box a random different colour is selected
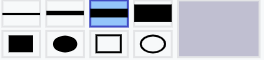
then at the very left bit of the header there is an image in the style of an old floppydisk when you click on this image the image drawn is downloaded to your device incase you grew emotionally attached to your work of art. these few lines seen below check if your mouse was clicked within a certain square and if it is the image is downloaded
if (collidebig((width / 128) * 2, height / 128)) {
saveCanvas("myCanvas", "jpg");
print("saving image");
in the code above the function “collidebig” is called, this is a function that checks whether or not a mouse was clicked within certain permitters of the given coordinate
function collidebig(x, y) {
if (
mouseX >= x &&
mouseX <= x + (width / 128) * 13 &&
mouseY >= y &&
mouseY <= y + (height / 128) * 13
) {
return true;
}
return false;
the Collide function does the same just within smaler parameters used to select colour or tools as shown below
if (collide((width / 128) * 58, height / 128)) {
x = 215;
y = 11;
z = 0;
now the last part is the extra stamp tools because of course noting is half assed or could ever be simpel. the stamp tool takes the x an d y coordinates and stufs them into arrays, these arrays are then used to draw the shapes
if (recty === true) {
if (point1 === undefined) {
if (mouseIsPressed) {
point1 = [mouseX, mouseY];
}
}
if (point1 != undefined) {
if (!mouseIsPressed) {
point2 = [mouseX, mouseY];
}
}
if (point1 != undefined && point2 != undefined) {
rectMode(CORNERS);
stroke(x, y, z);
fill(x, y, z);
rect(point1[0], point1[1], point2[0], point2[1]);
rectMode(CORNER);
point1 = undefined;
point2 = undefined;
}
}
if (circy === true) {
if (cpoint1 === undefined) {
if (mouseIsPressed) {
cpoint1 = [mouseX, mouseY];
}
}
if (cpoint1 != undefined) {
if (!mouseIsPressed) {
cpoint2 = [mouseX, mouseY];
}
}
if (cpoint1 != undefined && cpoint2 != undefined) {
ellipseMode(CORNERS);
stroke(x, y, z);
fill(x, y, z);
ellipse(cpoint1[0], cpoint1[1], cpoint2[0], cpoint2[1]);
cpoint1 = undefined;
cpoint2 = undefined;
}
that was it, 680 lines of code neatly stuffed and explained in over 700 words, not as bad as the last book i wrote for please touch everything